Naming Stuff is Hard: Why Proper Names Matter
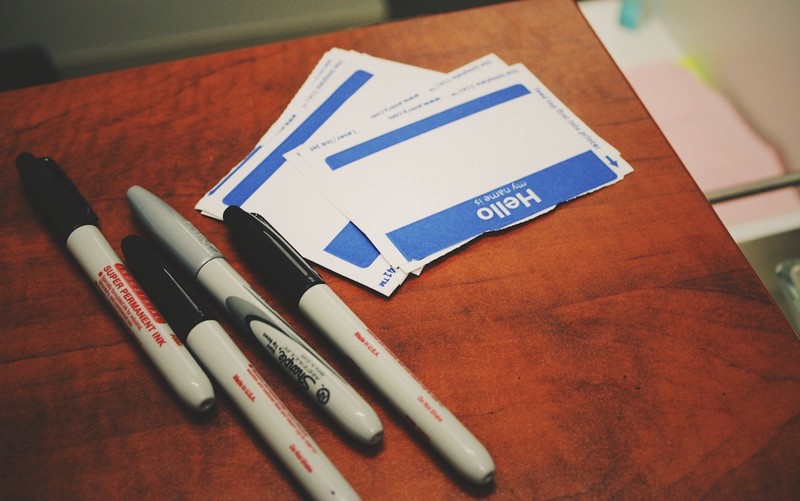
Good and clean code starts with proper names. It’s not easy to name every little thing in a concise and meaningful way, but it’s worth the effort.
With proper names, we can reduce the need for comments and make our code more easily understandable without any surprises. The reader of our code can focus on more important issues than weird, misleading, or non-topical names.
Table of Contents
Too short and too long
The names of our variables and types should answer questions about the code. Readability and self-explanation need to outweigh the convenience of less typing:
// weight in kg
long w;
versus
long weightKg;
No comment required.
The name clearly states the intent of the variable. Yes, the name is 8 times longer than before, but we have IDEs with auto-completion. So why make our code harder to read, just to save some keystrokes?
We all have i
or foo
or tmp
in our code.
But all variables deserve to be named.
Why not use int rowIdx
instead of int i
? Yes, it’s a few more keystrokes, but suddenly an incomprehensible loop index became meaningful.
You might know what the i
or tmp
means now, but are you sure to easily identify the meaning in a few months, days or even hours?
Sometimes this leads to the other extreme: Too long names.
We could have named the variable in our previous example customerCurrentWeightInKg
to tell an even bigger story about it.
But most likely, the surrounding context makes it not necessary to choose such a long name.
We should always be aware of the context and other surrounding information and shrink the name as much as possible, but still make it as long as necessary. If we can cut a word out, always cut it out. Prefer short and concise names and use longer names for when we have a good reason to do it.
We read code more often than we write it, so make it a pleasant read.
Conventions vs. Common Sense
Maybe the names AbstractInterceptorDrivenBeanDefinitionDecorator
, TransactionAwarePersistenceManagerFactoryProxy
, and CNLabelContactRelationYoungerCousinMothersSiblingsDaughterOrFathersSistersDaughter
are correctly defining what kind of objects they are, regarding the naming conventions of the project.
But they are horrible names… RequestProcessorFactoryFactory
is also correctly named, but still awful.
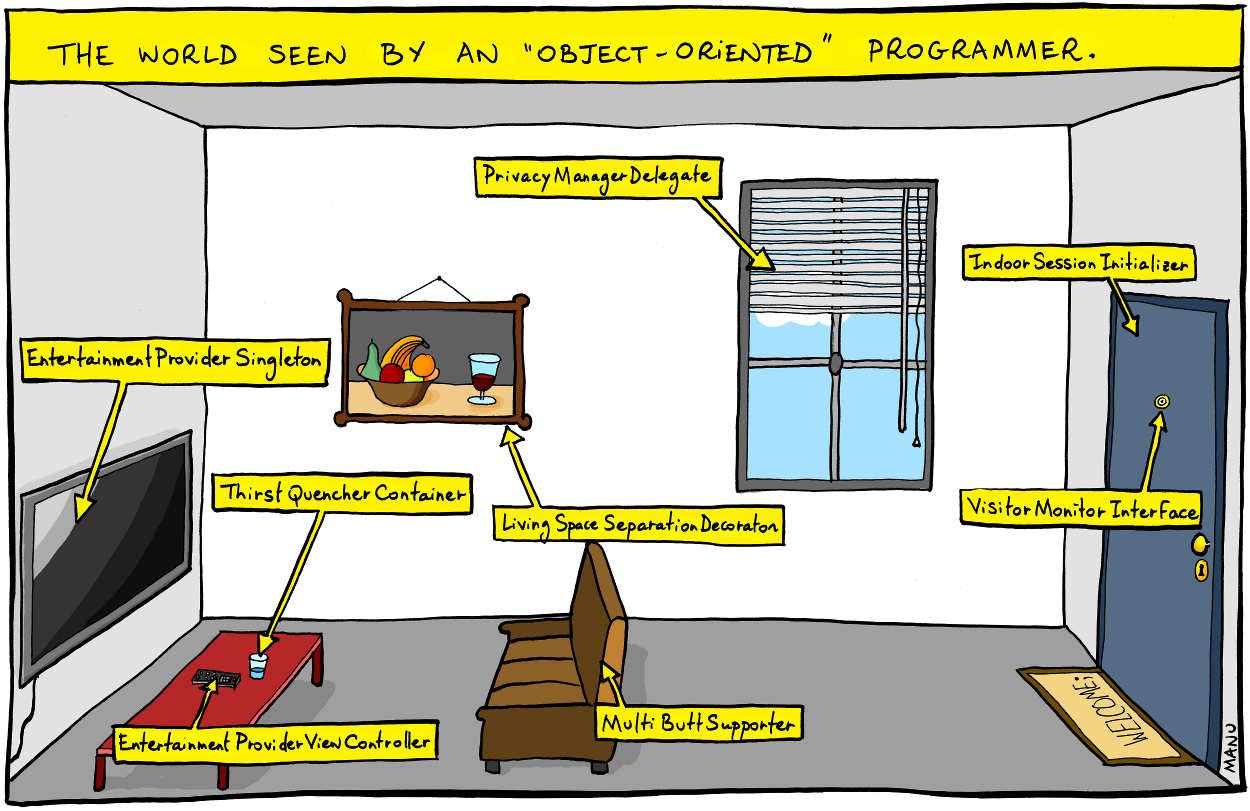
Always try to call things by what they really are, not what they are supposed to be, or what might sound smarter.
A CalendarManager
might actually just be a calendar, so why not call it Calendar
? Just like in real life, Managers
are often useless overhead, so try to avoid them.
The same goes for Controller
, Logic
, Service
, Provider
, etc.
Overusing design patterns might bleed into the naming of our code, and just being used to see these names in ours and others code doesn’t mean that you have to use these conventions all the time.
There might be good reasons to name certain classes in a specific way like only a Controller
is allowed to talk to the database, and a Service
only talks to a Controller
due to separation of concerns.
This way, the name carries contextual information and might be justified.
But be aware that such separations can quickly vanish or be misused, and our complicated naming scheme ends up doing only one thing: making things complicated.
Context is king
Our code lives in a certain domain with its own language and idioms. Try to name accordingly if fitting. Instead of simply using basic data-structures, we might want to encapsulate our code in a domain-specific way to be able to name things better:
// Bad: Simple List<String>
permissionsList.contains("admin:view");
// Better: Permission object
permissions.canView("admin");
Every software project has a common domain at its core. Use this domain to your advantage, not to restrict yourself. But don’t force unfitting names due to domain restrictions, common sense is also king. Like with all the other rules in software development, we need to find the balance not to overdo it.
One concept only needs one word
Especially in teams, it’s easy to end up using different words for the same concepts. It’s cause is manifold, be it different mother tongues amoung team members, cultural backgrounds, or different understanding of the concept itself.
One of the best examples is retrieving data, how would you call it?
- Get?
- Fetch?
- Lookup?
- Query?
- Retrieve?
- Find?
- Obtain?
- Collect?
All acceptable and proper words for the concept in general. But they nuance just a little bit, and the shades aren’t that clear if you ask different people.
Our team, and everyone involved in the code, should be able to grasp the general idea behind a concept by its name throughout our codebase.
So one word per concept is not just enough, it’s mandatory.
Choose the best name that doesn’t have too many meanings and doesn’t imply side effects not actually present in your code.
What is above all needed is to let the meaning choose the word_, and not the other way around. — George Orwell: How to name things
Bad names
Be meaningful: foo
vs. anything else
Be precise: data
vs. rawImageData
Be long enough: i
vs. rowIdx
Be short enough: customerCurrentWeightInKg
vs. weightKg
Be precise: DataManager
vs. CustomerDataManager
Don’t be funny: boringStuff
vs. anything else
Conclusion
Naming is one of the most important things in software development because it defines the understandability and maintainability of our code in the long run. It’s about communicating with our future self and our team, so choose your words wisely.
We should always try to write our code like if we have to maintain it for the rest of our lives.
If we are not able to name a variable correctly, we don’t know what it is. First and for all the name of our variables, types etc. should clearly answer two big questions:
- Why does it exist?
- What does it do?
And if it can’t answer these questions, we should try harder to find a better one.